Table of Contents
ToggleTop 75 Java Interview Questions for Freshers, Intermediates, and Experienced Candidates
Java remains one of the most popular programming languages, and mastering it can open doors to a plethora of career opportunities. Whether you’re a fresher just stepping into the world of Java development, an intermediate professional looking to level up, or a seasoned developer preparing for a senior role, it’s essential to be well-prepared for Java interviews. Below are 75 crucial Java interview questions categorized for freshers, intermediate, and experienced candidates, along with explanations to help you excel in your next interview.
—
Java Interview Questions for Freshers
As a fresher, Java interview questions are designed to assess your fundamental understanding of Java’s core concepts, syntax, and basic functionalities.
- What is Java?
– Java is a high-level, class-based, object-oriented programming language designed to have as few implementation dependencies as possible. It is widely used for building platform-independent applications.
- What are the features of Java?
– Some key features of Java include Object-Oriented, Platform-Independent, Secure, Robust, Portable, Multithreaded, and Distributed.
- What is the JDK, JRE, and JVM?
– JDK (Java Development Kit) is a software development kit to develop Java applications. JRE (Java Runtime Environment) is the environment in which Java bytecode can be executed. JVM (Java Virtual Machine) is an abstract machine that enables your computer to run a Java program.
- What is a Class in Java?
– A class in Java is a blueprint or prototype that defines objects. A class consists of variables, methods, and constructors.
- What is an Object in Java?
– An object is an instance of a class. It has state and behavior and is created using the `new` keyword.
- What are Java’s access specifiers?
– Java provides four types of access specifiers:
– `private`: Accessible only within the class.
– `default`: Accessible within the package.
– `protected`: Accessible within the package and by subclass.
– `public`: Accessible from anywhere.
- What is Inheritance in Java?
– Inheritance is a mechanism where one class inherits properties and behaviors (fields and methods) from another class.
- What is Polymorphism?
– Polymorphism allows objects to be treated as instances of their parent class. It includes method overloading (compile-time polymorphism) and method overriding (runtime polymorphism).
- What is Encapsulation?
– Encapsulation is the concept of wrapping data (variables) and methods (functions) into a single unit called class. It helps to achieve data hiding.
- What is Abstraction?
– Abstraction is the process of hiding the implementation details from the user and exposing only the essential functionalities. It is achieved using abstract classes and interfaces.
- What is a Constructor?
– A constructor in Java is a block of code used to initialize an object. It has the same name as the class and no return type.
- What is the difference between a Constructor and a Method?
– Constructors are used to initialize an object, while methods are used to perform functions. Constructors do not have a return type, while methods do.
- What is the ‘this’ keyword in Java?
– The `this` keyword refers to the current object in a method or constructor.
- What is the ‘super’ keyword?
– The `super` keyword refers to the immediate parent class object.
- What is Method Overloading?
– Method overloading is a feature in Java that allows a class to have more than one method with the same name, differentiated by their parameter lists.
- What is Method Overriding?
– Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its parent class.
- What is a Final Keyword?
– The `final` keyword in Java is used to declare constants, prevent method overriding, and prevent inheritance of a class.
- What is the difference between ‘==’ and ‘equals()’ method in Java?
– The `==` operator compares references, whereas the `equals()` method compares the values of objects.
- What is the purpose of the static keyword?
– The `static` keyword is used for memory management. It can be applied to variables, methods, blocks, and nested classes. Static members belong to the class rather than to any specific object.
- What is a Java Interface?
– An interface in Java is a reference type that contains abstract methods. A class implements an interface and provides method definitions for the interface methods.
- What is the difference between Abstract Class and Interface?
– An abstract class can have both abstract and concrete methods, while an interface can only have abstract methods (before Java 8). A class can inherit multiple interfaces but only one abstract class.
- What is the Java Collection Framework?
– The Collection Framework in Java is a set of classes and interfaces that helps manage groups of objects. It includes interfaces like List, Set, Map, and classes like ArrayList, HashSet, HashMap.
- What is an Array in Java?
– An array is a collection of similar types of elements stored in contiguous memory locations.
- What is Exception Handling in Java?
– Exception handling is a mechanism to handle runtime errors, allowing the normal flow of the application to continue. It uses `try`, `catch`, `finally`, and `throw`.
- What is the difference between Checked and Unchecked Exceptions?
– Checked exceptions are checked at compile time, while unchecked exceptions are checked at runtime.
Java Interview Questions for Intermediate Level
For intermediate-level Java developers, questions focus on advanced concepts like multithreading, collections, and design patterns.
- What is Multithreading in Java?
– Multithreading is the ability of a CPU or a single core to execute multiple threads simultaneously.
- What is the difference between a Process and a Thread?
– A process is an independent execution unit, while a thread is a smaller execution unit within a process.
- What is the `synchronized` keyword in Java?
– The `synchronized` keyword is used to control the access of multiple threads to a shared resource.
- What is Deadlock in Java?
– A deadlock occurs when two or more threads are blocked forever, each waiting on the other.
- What is a Thread Pool?
– A thread pool manages a pool of worker threads, which reduces the overhead of creating new threads every time for task execution.
- Explain the use of the `volatile` keyword in Java.
– The `volatile` keyword ensures that the value of a variable is always read from the main memory and not from a thread’s local cache.
- What is the Java Memory Model?
– The Java Memory Model defines how threads interact through memory and what behaviors are allowed in multithreaded environments.
- Explain the difference between `ArrayList` and `LinkedList`.
– `ArrayList` is a resizable array implementation, while `LinkedList` is a doubly linked list. `ArrayList` provides faster random access, and `LinkedList` provides faster insertions and deletions.
- What is a HashMap in Java?
– A HashMap is a collection that stores key-value pairs and provides constant-time performance for the basic operations (get and put).
- What is the difference between HashMap and HashTable?
– HashMap is non-synchronized and allows null values, whereas HashTable is synchronized and does not allow null values.
- What is a ConcurrentHashMap?
– ConcurrentHashMap is a thread-safe variant of HashMap that allows multiple threads to read and write without locking the entire map.
- What is the `Comparator` and `Comparable` interface in Java?
– The `Comparable` interface is used to define a natural ordering for objects, whereas the `Comparator` interface is used to define external comparison for objects.
- What is Serialization in Java?
– Serialization is the process of converting an object into a byte stream to store it in a file or transmit it over a network.
- What is Deserialization?
– Deserialization is the reverse process of serialization, where the byte stream is converted back into an object.
- What are the differences between `String`, `StringBuffer`, and `StringBuilder`?
– `String` is immutable, while `StringBuffer` and `StringBuilder` are mutable. `StringBuffer` is synchronized, and `StringBuilder` is not.
- What is Reflection in Java?
– Reflection is the ability of a program to examine and modify the runtime behavior of applications.
- What are Lambda Expressions in Java?
– Lambda expressions, introduced in Java 8, provide a way to write anonymous functions in a concise manner.
- What is Functional Interface?
– A functional interface is an interface that contains exactly one abstract method. They are used in lambda expressions.
- What is the Stream API in Java?
– The Stream API, introduced in Java 8, is used for processing collections of objects. It allows functional-style operations on streams of data.
- What are Optional in Java?
– `Optional` is a container object introduced in Java 8 that may or may not contain a non-null value, used to
avoid null pointer exceptions.
- What is the `default` method in Java interfaces?
– The `default` method allows adding new methods to interfaces without affecting classes that implement those interfaces.
- What is a Marker Interface?
– A marker interface does not contain any methods or fields and is used to mark a class with a specific behavior, like `Serializable`.
- What is the difference between deep and shallow copy in Java?
– A shallow copy copies only the object’s references, while a deep copy creates a new object and copies the content of the original object.
- Explain Dependency Injection in Java.
– Dependency Injection is a design pattern used to reduce the tight coupling between objects by injecting dependencies externally rather than creating them inside the class.
- What is a Singleton Class in Java?
– A singleton class allows only one instance of a class to be created and provides a global point of access to that instance.
Java Interview Questions for Experienced Developers
For experienced developers, the interview focuses on advanced topics, best practices, optimizations, and architectural designs.
- What are Design Patterns in Java?
– Design patterns are proven solutions to recurring problems in software design. Common design patterns include Singleton, Factory, Observer, and Decorator patterns.
- Explain the SOLID principles in Java.
– The SOLID principles are five design principles intended to make software designs more understandable, flexible, and maintainable.
– S: Single Responsibility Principle
– O: Open/Closed Principle
– L: Liskov Substitution Principle
– I: Interface Segregation Principle
– D: Dependency Inversion Principle
- What is Garbage Collection in Java, and how does it work?
– Garbage Collection is a process that automatically deallocates memory by identifying and removing objects that are no longer needed.
- What are the different types of Garbage Collectors in Java?
– Java provides several types of garbage collectors, such as Serial Garbage Collector, Parallel Garbage Collector, CMS (Concurrent Mark-Sweep), and G1 (Garbage First).
- Explain Java Memory Leaks and how to avoid them.
– A memory leak in Java occurs when an application unintentionally holds references to objects that are no longer used. Avoid memory leaks by carefully managing object references and using tools like heap dump analyzers.
- What are Soft, Weak, and Phantom References in Java?
– These are special types of references in Java that allow greater control over the object’s lifecycle in memory.
– Soft references: Objects are cleared only when memory is low.
– Weak references: Objects are cleared more aggressively.
– Phantom references: Objects are finalized and garbage collected but can be referenced for clean-up actions.
- What is a Memory Model in Java?
– The Java Memory Model defines the interactions of threads with memory and establishes rules for when changes to memory by one thread become visible to others.
- How do you optimize Java applications?
– Java applications can be optimized by:
– Efficient memory management.
– Reducing object creation.
– Using caching strategies.
– Using efficient data structures.
– Optimizing algorithms.
– Minimizing synchronization.
- Explain Microservices in Java.
– Microservices is an architectural style that structures an application as a collection of small, independent, and loosely coupled services. Each service is responsible for a specific business capability.
- What is Spring Framework in Java?
– Spring is a powerful Java framework used to build enterprise-level applications. It supports various features like Dependency Injection, Aspect-Oriented Programming, Data Access, and Web MVC.
- What is Hibernate in Java?
– Hibernate is a popular ORM (Object-Relational Mapping) framework that simplifies database interaction by mapping Java objects to database tables.
- Explain RESTful Web Services in Java.
– RESTful Web Services use HTTP protocol to create web services. REST (Representational State Transfer) allows interaction with web services using standard HTTP methods like GET, POST, PUT, DELETE.
- What is the difference between SOAP and REST?
– SOAP (Simple Object Access Protocol) is a protocol for exchanging structured information, while REST (Representational State Transfer) is an architectural style that uses HTTP for communication.
- What is Distributed Computing in Java?
– Distributed computing involves dividing a large application across multiple servers. Java supports distributed computing through technologies like RMI (Remote Method Invocation), EJB (Enterprise JavaBeans), and Web Services.
- What is a Microservice in Java, and how is it different from a Monolithic architecture?
– Microservices break down an application into independent services that communicate over a network, whereas monolithic architecture builds a single large application. Microservices offer greater flexibility and scalability.
- Explain how to scale Java applications.
– Java applications can be scaled by:
– Horizontal scaling (adding more instances).
– Vertical scaling (increasing resources of the instance).
– Efficient load balancing.
– Using a microservice architecture.
- What is Apache Kafka?
– Apache Kafka is a distributed messaging system that allows high-throughput, low-latency data streams for real-time analytics and event-driven architectures.
- How does Java handle large-scale concurrent systems?
– Java handles concurrency using its threading model, the concurrency API, Executor framework, and ForkJoin pools for efficient parallel processing.
- Explain the CAP Theorem in Java-based distributed systems.
– CAP theorem states that a distributed system can only provide two out of the following three guarantees:
– Consistency: Every read receives the most recent write.
– Availability: Every request receives a response.
– Partition Tolerance: The system continues to function despite network failures.
- What are Reactive Streams in Java?
– Reactive Streams allow asynchronous stream processing with non-blocking backpressure. Java has introduced Reactive Streams with frameworks like RxJava and Project Reactor.
- What is Docker, and how is it used in Java development?
– Docker is a platform for building, deploying, and managing containers. In Java development, Docker helps in containerizing Java applications, ensuring consistency across development and production environments.
- What are Kubernetes and its use in Java?
– Kubernetes is an open-source container orchestration system. It manages containers (like those built using Docker) and helps in scaling Java applications efficiently in a cloud environment.
- How does JVM performance tuning work?
– JVM performance tuning involves adjusting memory settings, garbage collection parameters, and runtime flags to optimize the execution of Java applications.
- What is Aspect-Oriented Programming (AOP)?
– AOP is a programming paradigm that allows the separation of cross-cutting concerns, such as logging and security, from the business logic of a Java application.
- Explain the Circuit Breaker pattern.
– The Circuit Breaker pattern prevents an application from repeatedly trying to execute an operation that’s likely to fail, such as a network call, to avoid cascading failures in distributed systems.
Conclusion
Preparing for a Java interview requires a deep understanding of core concepts, advanced features, and architectural patterns. The questions above cover a wide range of topics from basic Java fundamentals to more advanced concepts for intermediate and experienced candidates. Whether you’re just starting out or looking to progress in your career, these questions will help you demonstrate your knowledge and ace your next Java interview.
Good luck
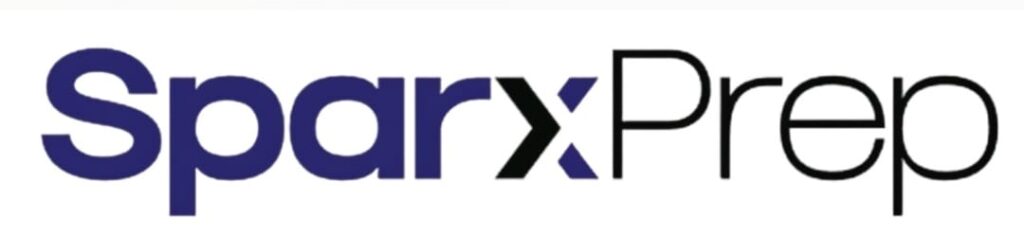
Hey harsh my harsh batchmate